RPA is a way of automating processes that do not have the use of an API. RPA can be used to automate repetitive tasks on; websites or desktop applications.
I thought this would be a good way of adding IP addresses to my Scrapli & Flask Project without using an API (as I haven’t added on yet).
I have used RPA for Python which can be found here: https://github.com/tebelorg/RPA-Python
The below installation guide and sample scripts should be enough to get this setup and tested.
Installation: Windows
I had a number of problems when installing and getting it to run first time on Windows. So I hope this is of some use.
0 1 2 |
pip install rpa |
Error 1: TagUI Not Installed
TagUI was not being correctly installed. As I understand it, is what the Python package uses as its API. If this was not installed then it cannot work.
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
[RPA][INFO] - setting up TagUI for use in your Python environment [RPA][INFO] - downloading TagUI (~200MB) and unzipping to below folder... [RPA][INFO] - C:\Users\Stef\AppData\Roaming [RPA][INFO] - done. syncing TagUI with stable cutting edge version [RPA][INFO] - now installing missing Visual C++ Redistributable dependency 'C:\Users\Stef\AppData\Roaming/tagui/vcredist_x86.exe' is not recognized as an internal or external c operable program or batch file. [RPA][INFO] - the vcredist_x86.exe file in C:\Users\Stef\AppData\Roaming\tagui or from [RPA][INFO] - https://www.microsoft.com/en-us/download/details.aspx?id=30679 [RPA][INFO] - after that, TagUI ready for use in your Python environment 'C:\Users\Stef\AppData\Roaming/tagui/src/end_processes' is not recognized as an internal or external operable program or batch file. [RPA][ERROR] - following happens when starting TagUI... The following command is executed to start TagUI - "C:\Users\Stef\AppData\Roaming/tagui/src/tagui" rpa_python chrome It leads to following output when starting TagUI - 'C:\Users\Stef\AppData\Roaming/tagui/src/tagui' is not recognized as an internal or external command, operable program or batch file. [RPA][ERROR] - unknown error encountered [RPA][ERROR] - use init() before using url() [RPA][ERROR] - use init() before using type() [RPA][ERROR] - use init() before using read() [RPA][ERROR] - use init() before using snap() [RPA][ERROR] - use init() before using close() |
RPA does try to download and install the TagUI software in the location C:\Users\USERNAME\AppData\Roaming.
However this did not work for me and I needed to manually install it.
https://github.com/tebelorg/TagUI#set-up
Select the version you are using. My case it was Windows.
I then extracted the file to C:\Users\USERNAME\AppData\Roaming\tagui
The directory structure should be: C:\Users\USERNAME\AppData\Roaming\tagui\src\tagui.cmd
This will be important later!
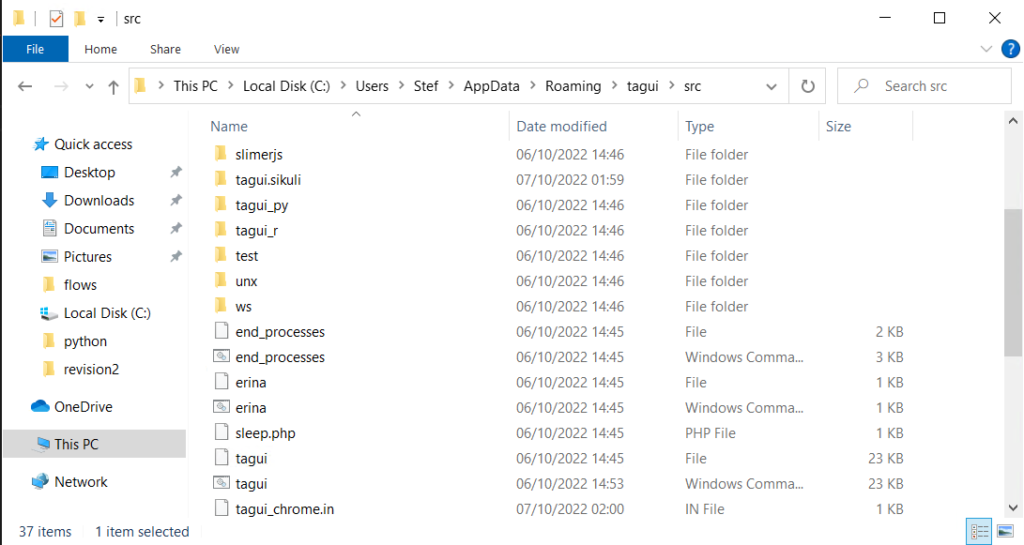
Error 2: Chrome in Wrong Location
Chrome default location for installation is: C:\Program Files\Google\Chrome\Application\chrome.exe
TagUI had it in C:\Program Files (x86). This gave me a similar error to the one above.
0 1 2 3 4 |
It leads to following output when starting TagUI - ERROR - cannot find Chrome at "C:\Program Files (x86)\Google\Chrome\Application\chrome.exe" update chrome_command setting in tagui\src\tagui.cmd to your chrome.exe |
To resolve I just updated the location in the tagui.cmd
This is why it is important to have the paths all correct when installing manually.
0 1 2 3 4 |
Update Location in file: C:\Users\Stef\AppData\Roaming\tagui\src\tagui.cmd set chrome_command=C:\Program Files\Google\Chrome\Application\chrome.exe |
Now web automation for rpa should work. Test it out!
0 1 2 3 4 5 6 7 8 9 10 11 12 13 |
import rpa as r import os basedir = os.path.abspath(os.path.dirname(__file__)) file_save = (basedir + "\\webtest.png") r.init() r.url('https://www.google.com') r.type('//*[@name="q"]', 'web automation example[enter]') print(r.read('result-stats')) r.snap('page', file_save) r.close() |
Error 3: Install JDK
Now the main application is installed, we need to add in the additional JDK software too. this will allow for visual automation such as mouse/keyboard automation.
Windows download: https://corretto.aws/downloads/latest/amazon-corretto-8-x64-windows-jdk.msi
0 1 2 3 4 5 6 7 |
[RPA][INFO] - to use visual automation mode, OpenJDK v8 (64-bit) or later is required [RPA][INFO] - download from Amazon Corretto's website - https://aws.amazon.com/corretto [RPA][INFO] - OpenJDK is preferred over Java JDK which is free for non-commercial use only [RPA][ERROR] - use init() before using keyboard() [RPA][ERROR] - use init() before using keyboard() [RPA][ERROR] - use init() before using close() |
Once installed try to run a script. On my first run I did receive an error similar to the one below.
Neither jython nor jruby available, IDE not yet usable with JavaScript only

After running this a second time, no more error everything worked fine.
0 1 2 3 4 5 6 7 8 9 10 11 12 13 |
import rpa as r import os basedir = os.path.abspath(os.path.dirname(__file__)) file_save = (basedir + "\\keyboardtest.png") r.init() r.url('https://www.google.com') r.wait(2.5) r.click('//*[text() = "Sign in"]') r.wait(10) r.close() |