This is a quick note of how to create buttons that can toggle forms using jQuery. I used this in the F5 Dashboard to display different forms.
The user can click on the top or bottom to reveal that. Then clicking the other button will hide the first to only reveal the second.
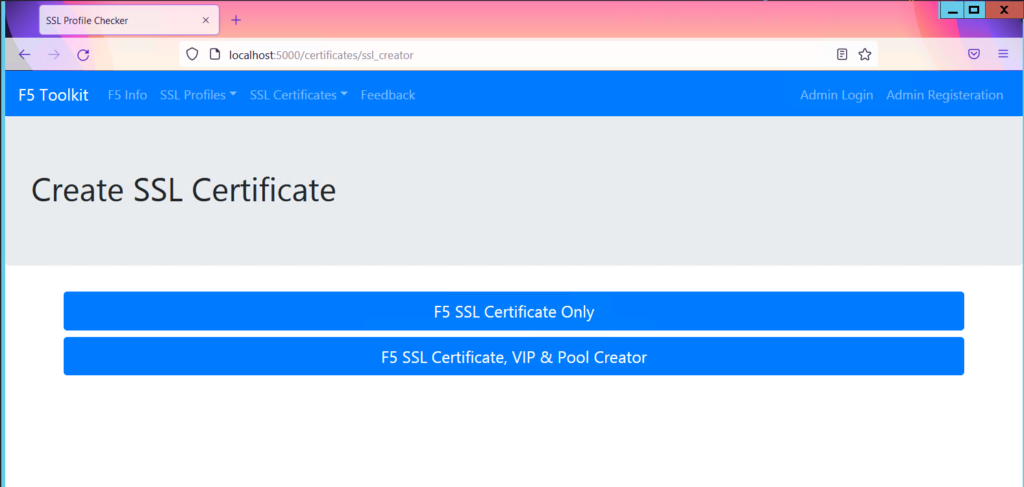
Example JSFiddle
I have a jsfiddle.net example that is useful for demonstration purposes of toggling a form with a button.
https://jsfiddle.net/pairdocs/3bxq1s6a/20/
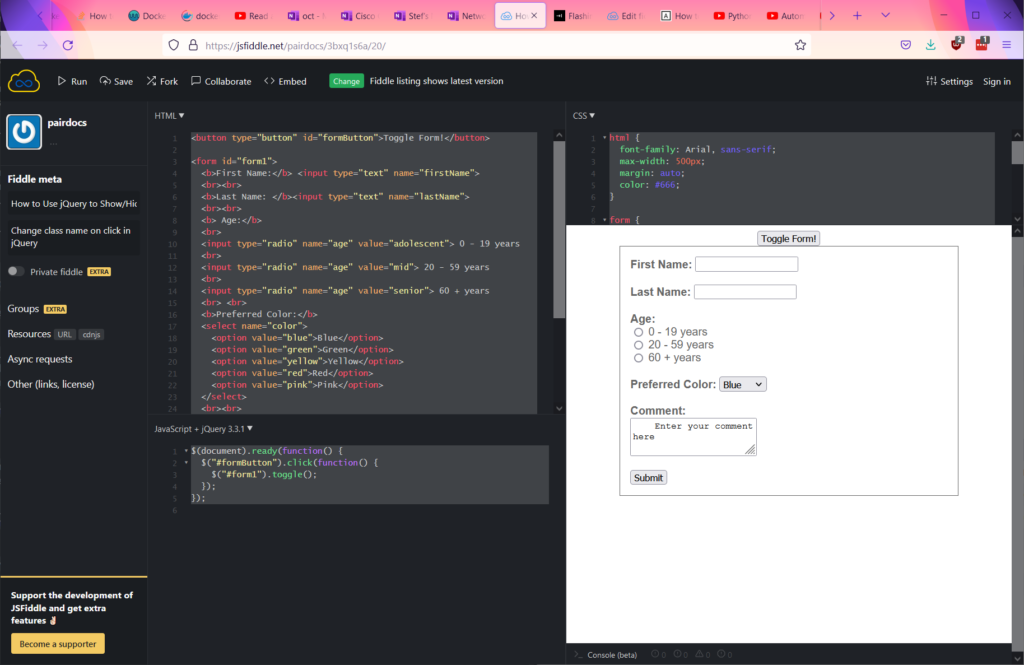
HTML
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
<button type="button" id="formButton">Toggle Form!</button> <form id="form1"> <b>First Name:</b> <input type="text" name="firstName"> <br><br> <b>Last Name: </b><input type="text" name="lastName"> <br><br> <b> Age:</b> <br> <input type="radio" name="age" value="adolescent"> 0 - 19 years <br> <input type="radio" name="age" value="mid"> 20 - 59 years <br> <input type="radio" name="age" value="senior"> 60 + years <br> <br> <b>Preferred Color:</b> <select name="color"> <option value="blue">Blue</option> <option value="green">Green</option> <option value="yellow">Yellow</option> <option value="red">Red</option> <option value="pink">Pink</option> </select> <br><br> <b>Comment:</b> <br> <textarea name="comment"> Enter your comment here </textarea> <br><br> <button type="button" id="submit">Submit</button> </form> |
jQuery
0 1 2 3 4 5 6 |
$(document).ready(function() { $("#formButton").click(function() { $("#form1").toggle(); }); }); |
CSS
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
html { font-family: Arial, sans-serif; max-width: 500px; margin: auto; color: #666; } form { padding: 15px; border: 1px solid #666; background: #fff; display: none; } #formButton { display: block; margin-right: auto; margin-left: auto; } |
F5 Dashboard
The F5 Dashboard is slightly different to the jsfiddle.net example as it has two buttons.
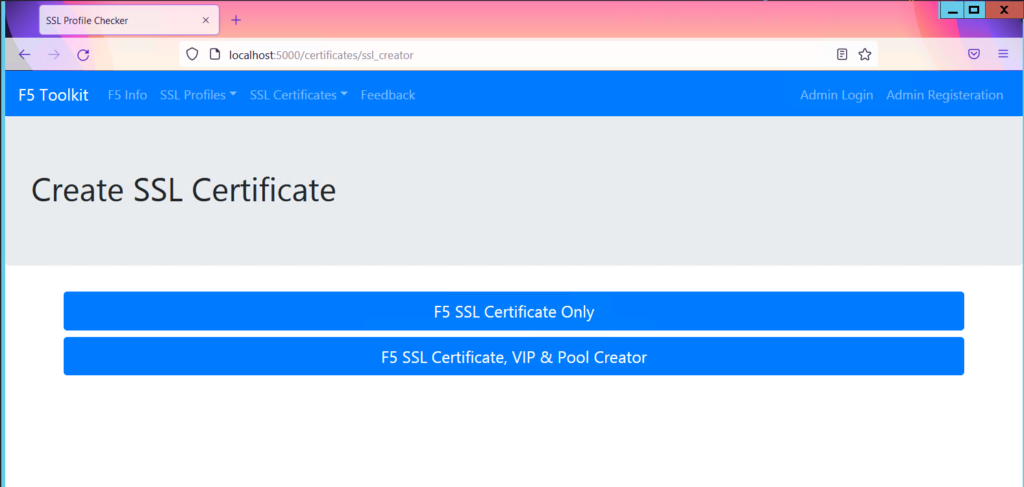
HTML
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 |
{% extends "base.html" %} {% block content %} <div class="jumbotron"> <h1>Create SSL Certificate</h1> </div> <div class='container'> <button type="button" class="btn btn-primary btn-lg btn-block" id="buttonSSLonly">F5 SSL Certificate Only</button> <button type="button" class="btn btn-primary btn-lg btn-block" id="buttonSSLcomplete">F5 SSL Certificate, VIP & Pool Creator</button> <br> <form id="formSSLonly" method="POST"> <h3>F5 SSL Certificate Only</h3> {{ formSSLonly.hidden_tag() }} {{ formSSLonly.domain_name.label }}{{ formSSLonly.domain_name (class="form-control", placeholder="Enter domain name here, e.g. useradmin.uk.staging.ehc.test.com") }} <br> {{ formSSLonly.sans.label }}{{ formSSLonly.sans (class="form-control", placeholder="Enter domain name here, e.g. example.test.com or *.test.com", id="floatingTextarea2", style="height: 75px") }} <br> {{ formSSLonly.mgmt_ip.label }}{{ formSSLonly.mgmt_ip (class="form-control", placeholder="Enter F5 management IP") }} <br> {{ formSSLonly.submit(class="btn btn-primary") }} </form> <form id="formSSLcomplete" method="POST"> <h3>F5 SSL Certificate, VIP & Pool Creator</h3> {{ formSSLcomplete.hidden_tag() }} {{ formSSLcomplete.service_name.label }}{{ formSSLcomplete.service_name (class="form-control", placeholder="Enter service here, e.g. SSL-Service1") }} <br> {{ formSSLcomplete.domain_name.label }}{{ formSSLcomplete.domain_name (class="form-control", placeholder="Enter domain name here, e.g. useradmin.uk.staging.ehc.test.com") }} <br> {{ formSSLcomplete.sans.label }}{{ formSSLcomplete.sans (class="form-control", placeholder="Enter domain name here, e.g. example.test.com or *.test.com", id="floatingTextarea2", style="height: 75px") }} <br> {{ formSSLcomplete.ip.label }}{{ formSSLcomplete.ip (class="form-control", placeholder="Enter IP here") }} <br> {{ formSSLcomplete.rd.label }}{{ formSSLcomplete.rd (class="form-control", placeholder="Enter route domain here") }} <br> {{ formSSLcomplete.port.label }}{{ formSSLcomplete.port (class="form-control", placeholder="Enter port here e.g. 443") }} <br> {{ formSSLcomplete.shape_reqd.label }}{{ formSSLcomplete.shape_reqd (class="form-check") }} <br> {{ formSSLcomplete.mgmt_ip.label }}{{ formSSLcomplete.mgmt_ip (class="form-control", placeholder="Enter F5 management IP") }} <br> {{ formSSLcomplete.pool_members.label }}{{ formSSLcomplete.pool_members (class="form-control", placeholder="Enter F5 pool member IP and ports, separated by a comma e.g. 2.2.2.2:80,3.3.3.3:80", id="floatingTextarea2", style="height: 75px") }} <br> {{ formSSLcomplete.redirect_reqd.label }}{{ formSSLcomplete.redirect_reqd (class="form-check") }} <br> {{ formSSLcomplete.submit(class="btn btn-primary") }} </form> </div> <script src="{{ url_for('static', filename='js/myfile.js')}}"></script> {% endblock %} html { /* font-family: Arial, sans-serif; */ max-width: auto; /* color: #666; */ } #formSSLonly { display: none; } #formSSLcomplete { display: none; } #formButton1 { display: block; /* margin-right: auto; margin-left: auto; */ } #formButton2 { display: block; float: right; /* margin-right: auto; margin-left: auto; */ } |
jQuery
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
$(document).ready(function() { $("#buttonSSLonly").click(function() { $("#formSSLonly").toggle(); $("#formSSLcomplete").hide(); }); $("#buttonSSLcomplete").click(function() { $("#formSSLcomplete").toggle(); $("#formSSLonly").hide(); }); }); |
CSS
0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
html { /* font-family: Arial, sans-serif; */ max-width: auto; /* color: #666; */ } #formSSLonly { display: none; } #formSSLcomplete { display: none; } #formButton1 { display: block; /* margin-right: auto; margin-left: auto; */ } #formButton2 { display: block; float: right; /* margin-right: auto; margin-left: auto; */ } |